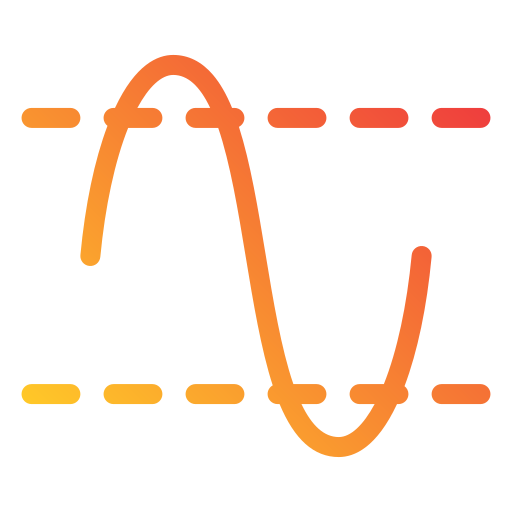
Quantum Signal processing
Signal processing is the field of study that deals with analyzing, modifying, and interpreting signals. Signals are electrical or acoustic representations of phenomena that vary over time or space. Examples of signals include sound waves, images, and electromagnetic waves.
The utilization of machine learning techniques for solving signal processing problems has a long history. Early machine learning applications to signal processing included speech recognition and image classification, where neural networks were used to model complex non-linear relationships between the input and output signals. More recently, deep learning techniques have been applied to a wide range of signal processing problems, including image and speech processing, radar and sonar signal processing, and wireless communications.
Quantum Signal Processing (QSP) is a relatively new field at the intersection of quantum information theory and signal processing. The goal of quantum signal processing is to develop new signal processing techniques that can exploit the unique properties of quantum systems, such as superposition and entanglement, to improve the performance of classical signal processing systems. It involves the development of algorithms and techniques that use quantum mechanics to process and analyze signals.
There is no single inventor of quantum signal processing, as it is a rapidly evolving and interdisciplinary field with contributions from many researchers. However, some notable pioneers in the field include Seth Lloyd, John Preskill, and Peter Shor, who have significantly contributed to developing quantum algorithms and quantum error correction codes.
One reason why quantum signal processing could be a good tool to improve classical signal processing is that it offers the potential for exponential speedup over classical algorithms for certain tasks. For example, quantum algorithms like Grover’s and Shor’s algorithms have been shown to provide significant speedup over classical algorithms for searching and factoring problems, respectively.
In addition to speedup, quantum signal processing can also provide new capabilities that are not possible with classical signal processing. For example, quantum entanglement allows for generating correlated signals that could be useful in secure communication and distributed sensing applications.
One of the main differences between classical and quantum signal processing is that in quantum signal processing, signals are represented using quantum bits, or qubits, rather than classical bits. Qubits can be in a superposition of states, allowing for representing more complex signals than classical bits. Additionally, quantum systems can be entangled, which means that the state of one qubit can be dependent on the state of another qubit, even if they are physically separated. This property can be exploited to develop novel signal-processing techniques that are otherwise impossible.
Quantum signal processing has the potential to improve classical signal processing in several ways. For example, quantum algorithms can perform Fourier transforms much faster than classical algorithms, leading to faster signal processing. Additionally, quantum algorithms can be used to perform certain types of optimization problems more efficiently than classical algorithms, which can be useful in a wide range of signal-processing applications. Finally, quantum communication protocols can be used to transmit information more securely than classical communication protocols, which can be useful in applications where data privacy is critical.
Overall, quantum signal processing is an emerging field that has the potential to revolutionize signal processing by exploiting the unique properties of quantum systems. While the field is still in its infancy, there has been significant progress in developing new algorithms and techniques for quantum signal processing, and it is likely that quantum signal processing will play an increasingly important role in signal processing in the future.
Here’s an example that uses quantum machine learning to classify audio signals:
# Import PennyLane and NumPy
import pennylane as qml
import numpy as np
# Define the quantum circuit
dev = qml.device('default.qubit', wires=2)
@qml.qnode(dev)
def circuit(params, x):
# Encode the signal using rotation gates
qml.Rot(*params[0], wires=0)
qml.Rot(*params[1], wires=1)
# Apply the SWAP test to measure the similarity of the signals
qml.Hadamard(wires=2)
qml.CSWAP(wires=[2,0,1])
qml.Hadamard(wires=2)
return qml.expval(qml.PauliZ(2))
# Define the cost function for training the model
def cost(params, x, y):
loss = 0
for i in range(len(x)):
prediction = circuit(params, x[i])
loss += (prediction - y[i])**2
return loss / len(x)
# Generate some example signals and labels
x = np.array([[0.1, 0.2], [0.3, 0.4], [0.5, 0.6], [0.7, 0.8]])
y = np.array([-1, 1, 1, -1])
# Train the quantum model using gradient descent
params = np.random.rand(2,3)
learning_rate = 0.1
num_epochs = 100
for epoch in range(num_epochs):
params -= learning_rate * qml.grad(cost)(params, x, y)
loss = cost(params, x, y)
print(f"Epoch {epoch+1}: loss = {loss}")
# Classify a new signal using the trained model
new_signal = np.array([0.9, 1.0])
prediction = circuit(params, new_signal)
print(f"Prediction for new signal: {prediction}")
In this example, the quantum circuit encodes the audio signals as rotations on two qubits and then applies the SWAP test to measure the similarity of the signals. The resulting quantum state is measured using a Pauli-Z measurement on the third qubit. The cost function measures the difference between the predicted and actual labels, and the model is trained using gradient descent.
A practical use case for this type of quantum signal processing could be in audio or speech recognition, where the goal is to classify audio signals based on their content or speaker. By using quantum machine learning techniques, it may be possible to improve the accuracy of these classification tasks, especially for signals that are difficult to analyze using classical signal processing methods.