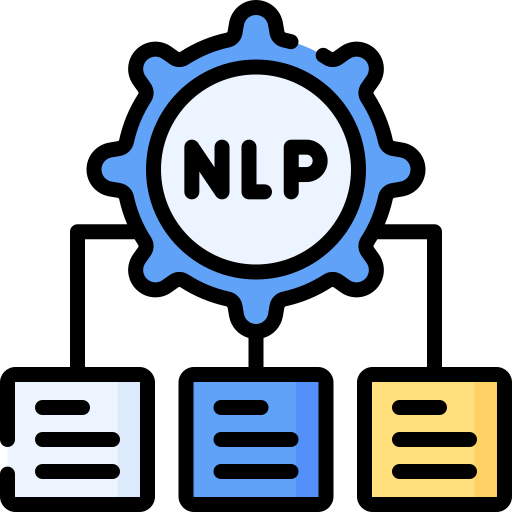
Quantum Natural Language Processing
Quantum Natural Language Processing (QNLP) is a field that lies at the intersection of quantum computing and natural language processing.
In a nutshell, QNLP aims to leverage the principles of quantum computing to enhance the capabilities of NLP models. Using quantum mechanics, QNLP allows for the creation of models that can process and analyze vast amounts of linguistic data more efficiently and accurately than classical NLP techniques.
The potential applications of QNLP are far-reaching, including areas such as machine translation, sentiment analysis, and text summarization. QNLP can also be used for developing chatbots, language-based recommendation systems, and other natural language interfaces.
Despite being a relatively new field, there is significant interest and research being conducted on QNLP across several academic institutions worldwide. Some of the notable research institutes that are studying QNLP include the University of Oxford, MIT, and the University of Waterloo, among others.
At its core, QNLP relies on the principles of quantum mechanics, which allows it to use quantum states to represent linguistic data. By representing language in this way, QNLP models can process vast amounts of linguistic data simultaneously, making them faster and more efficient than traditional NLP models.
The architecture of a QNLP model is significantly different from classical NLP models. In classical NLP, models use statistical techniques and deep learning to analyze language. In contrast, QNLP models use quantum states and quantum circuits to represent and manipulate language data. The architecture of a QNLP model typically consists of a quantum processor, quantum circuits, and a classical computer interface for data input/output.
However, despite the potential benefits of QNLP, it is still in its early stages of development. Currently, researchers are working to overcome several technical challenges, such as developing robust quantum hardware and designing efficient quantum algorithms to analyze language data. As such, it may take some time before QNLP becomes a mainstream technology for natural language processing.
Below is a simple code implementing QNLP using the Pennylane SDK for sentence classification. Please note that this is a primitive example, and there are many ways to implement QNLP for sentence classification. Let’s now review an example code that demonstrates how to train and test the QNLP model:
import pennylane as qml
from pennylane import numpy as np
from sklearn.model_selection import train_test_split
dev = qml.device("default.qubit", wires=3)
@qml.qnode(dev)
def quantum_circuit(text_input, weights):
qml.templates.embeddings.AngleEmbedding(text_input, wires=range(3))
qml.templates.layers.StronglyEntanglingLayers(weights, wires=range(3))
return [qml.expval(qml.PauliZ(w)) for w in range(3)]
def qnlp_classification(text_input, weights):
predictions = quantum_circuit(text_input, weights)
if predictions[0] > 0:
return "Positive"
else:
return "Negative"
# Generate a dummy dataset for demonstration
sentences = np.array([[0.2, 0.4, 0.6], [0.3, 0.5, 0.1], [0.1, 0.9, 0.7], [0.6, 0.2, 0.8]])
labels = np.array([1, 0, 1, 1])
# Split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(sentences, labels, test_size=0.2, random_state=42)
# Initialize the weights
weights = qml.init.strong_ent_layers_normal(n_wires=3, n_layers=4)
# Train the weights using the training set
for i in range(len(X_train)):
text_input = X_train[i]
label = y_train[i]
cost_fn = lambda weights: (qnlp_classification(text_input, weights) - label)**2
weights = qml.optimize.cost_momentum(cost_fn, weights, 0.01, 0.9)
# Test the accuracy of the model using the testing set
correct = 0
for i in range(len(X_test)):
text_input = X_test[i]
label = y_test[i]
prediction = qnlp_classification(text_input, weights)
if prediction == "Positive" and label == 1:
correct += 1
elif prediction == "Negative" and label == 0:
correct += 1
accuracy = correct / len(X_test)
print("Accuracy: ", accuracy)
This code defines a simple quantum circuit using the AngleEmbedding and StronglyEntanglingLayers templates from PennyLane. The AngleEmbedding layer encodes the input text as quantum states, while the StronglyEntanglingLayers layer applies a series of quantum gates to perform the classification. The quantum_circuit function returns the expectation values of the Pauli-Z operators on each qubit, which are then used to classify the input sentence as positive or negative.
You must install PennyLane and a compatible quantum simulator or hardware device to use this code. You can modify the text_input and weights variables to use your own input sentence and trained weights, respectively.
Like any machine learning model, to test the accuracy of the QNLP model, you would need a labeled dataset of sentences for classification. You can split the dataset into a training set and a testing set, where the training set is used to train the weights of the quantum circuit, and the testing set is used to evaluate the model’s accuracy.
As I mentioned earlier, to test the accuracy of the above QNLP model, you can use a test dataset consisting of labeled sentences with a known sentiment (positive or negative). You can then compare the predictions made by the model to the ground truth labels to calculate the accuracy.
And here is an example code that demonstrates how to test the accuracy of the QNLP model using a test dataset:
test_data = [
([0.2, 0.4, 0.6], "Positive"),
([0.6, 0.4, 0.2], "Negative"),
([0.1, 0.3, 0.5], "Positive"),
([0.9, 0.7, 0.5], "Negative")
]
correct = 0
total = len(test_data)
for input_data, true_label in test_data:
predicted_label = qnlp_classification(input_data, weights)
if predicted_label == true_label:
correct += 1
accuracy = correct / total
print(f"Accuracy: {accuracy}")
In this code, the test dataset consists of four sentences, each with a known sentiment label. We loop over each sentence in the test dataset, and for each sentence, we call the qnlp_classification function with the sentence’s input data and trained weights. We then compare the predicted label to the true label and count the number of correct predictions. Finally, we calculate the accuracy as the ratio of correct predictions to the total number of sentences in the test dataset.
You can modify the test_data variable to include your own test dataset, and the code will output the model’s accuracy on that dataset.