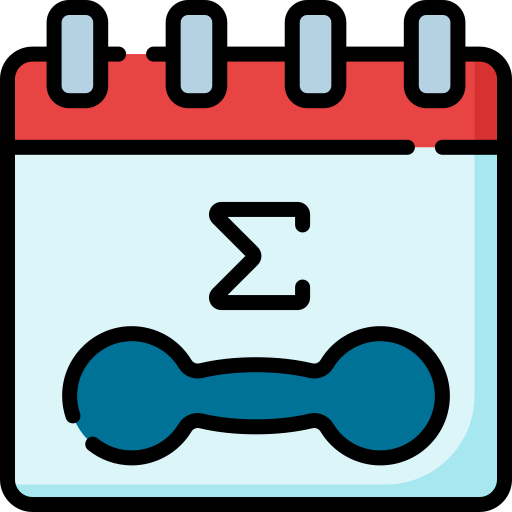
employee shift scheduling
Quantum annealing is a type of quantum computation that is specifically designed to solve optimization problems. Optimization problems require finding the minimum or maximum value of an objective function with respect to a set of variables.
Quantum annealing works by using a quantum mechanical phenomenon called quantum tunneling, which enables a quantum annealer to escape local minima and find the global minimum of the objective function. The quantum annealer starts in a state where all qubits are initially in a superposition of states. Then it slowly anneals the system from this initial state to a final state that encodes the solution to the optimization problem.
To solve an optimization problem with a quantum annealer, the problem must first be encoded as an Ising model or a Quadratic Unconstrained Binary Optimization (QUBO) problem. The Ising model is a mathematical model that describes the interactions between magnetic spins on a lattice, while QUBO is a type of optimization problem that involves finding the minimum value of a quadratic polynomial.
Quantum annealing can solve various optimization problems, including those that arise in materials science, finance, logistics, and machine learning. For example, it can be used to find the optimal configuration of a protein’s amino acids, optimize portfolio investments, or route delivery trucks to minimize travel time.
D-Wave Systems is currently the leading company in the quantum annealing industry, and it has developed several quantum annealing processors available for commercial use. However, it is worth noting that other companies, such as IBM and Google, are also developing quantum annealing technologies and exploring their potential applications.
Quantum annealing and adiabatic quantum computing are related approaches to solving optimization problems using quantum mechanical systems, but there are some important differences between the two.
In quantum annealing, the system starts in a superposition of states and evolves slowly to a final state representing the solution to an optimization problem. The system’s Hamiltonian drives the evolution, which encodes the problem’s objective function. During the annealing process, the system is constantly monitored to ensure that it remains in its ground state.
Adiabatic quantum computing (AQC) is similar to quantum annealing in that it also uses a slowly evolving Hamiltonian to find the solution to an optimization problem. However, in AQC, the system starts in the ground state of a known Hamiltonian, which is easy to prepare, and then slowly evolves to the ground state of the problem Hamiltonian. The evolution is adiabatic, meaning that the system stays in its ground state at all times, and the final state encodes the solution to the optimization problem.
As explained, the main difference between quantum annealing and adiabatic quantum computing is the starting state of the system. In quantum annealing, the system starts in a superposition of states, which is more difficult to prepare and monitor than the ground state used in AQC. However, quantum annealing can be more efficient for certain types of problems, particularly those that have a sparse connectivity structure.
In contrast, AQC is more flexible and can be applied to a broader range of problems, including those that are not amenable to quantum annealing. Additionally, AQC is a more general approach to quantum computation, whereas quantum annealing is specialized for optimization problems.
Overall, quantum annealing and adiabatic quantum computing are two related but distinct approaches to quantum optimization, with their own advantages and limitations.
Well, let’s now keep our focus on an interesting optimization use case. Are you tired of spending countless hours manually scheduling employee shifts? Do you struggle to find an optimal schedule that meets all your business requirements while keeping your employees happy? If so, quantum annealing might be the solution you need!
Quantum annealing is a cutting-edge technology that leverages the power of quantum mechanics to find the best solutions to complex optimization problems. And one area where quantum annealing can be particularly useful is employee shift scheduling.
Using a quantum annealer, you can encode your business requirements and employee preferences into an optimization problem, which the annealer can solve in a fraction of the time (microseconds) it would take a classical computer. The result is an optimal shift schedule that meets all your needs and satisfies your employees.
But how exactly does this work? Let’s take a look at some code to see quantum annealing in action for employee shift scheduling. Below is a Python program that uses the D-Wave quantum annealer through the LeapHybridDQMSampler to solve a classical optimization problem.
The problem involves employee scheduling. There are four shifts, and each of the four employees has a preference for which shift they would like to work. The goal is to assign each employee to a shift in such a way as to minimize the overall dissatisfaction among the employees, given their individual preferences.
Then we defined several functions to create a Discrete Quadratic Model (DQM) and to solve the optimization problem using a DQM solver. The employee_preferences() function defines each employee’s preferences as a dictionary.
The build_dqm() function creates a DQM object and adds variables for each employee, with each variable corresponding to a shift. It then uses the preferences dictionary to set linear weights on the variables. The solve_problem() function uses the DQM object and the LeapHybridDQMSampler to find a solution to the optimization problem.
Lastly, the build_dqm(), set_sampler(), and solve_problem() functions are called to solve the problem. The first solution found by the solver will be printed, along with the corresponding energy. Finally, the solution is interpreted in terms of the shifts assigned to each employee.
from dimod import DiscreteQuadraticModel
from dwave.system import LeapHybridDQMSampler
class EmployeeScheduler:
def __init__(self):
self.preferences = {"Jennifer": [1, 2, 3, 4],
"Will": [3, 2, 1, 4],
"Adam": [4, 2, 3, 1],
"Rebecca": [4, 1, 2, 3]}
self.num_shifts = 4
self.shifts = [1, 2, 3, 4]
self.dqm = DiscreteQuadraticModel()
self.sampler = LeapHybridDQMSampler()
def set_token(self, token):
'''Sets the personal access token.'''
self.token = token
def set_sampler(self):
'''Sets the dimod sampler.'''
self.sampler = LeapHybridDQMSampler()
def build_dqm(self):
'''Builds the DQM for the employee scheduling problem.'''
for name in self.preferences:
self.dqm.add_variable(self.num_shifts, label=name)
for name in self.preferences:
self.dqm.set_linear(name, self.preferences[name])
def solve_problem(self):
'''Runs the DQM on the designated sampler.'''
self.sampleset = self.sampler.sample_dqm(self.dqm, label='Training - Employee Scheduling')
def print_schedule(self):
'''Prints the first solution of the employee scheduling problem.'''
sample = self.sampleset.first.sample
energy = self.sampleset.first.energy
print(sample, energy, "\n")
for key, val in sample.items():
print("Schedule", key, "for shift", self.shifts[val])
And here is a driver code to run the application on D-wave QPU.
scheduler = EmployeeScheduler()
scheduler.set_token('XXXXX')
scheduler.build_dqm()
scheduler.solve_problem()
scheduler.print_schedule()
In summary, in the above code, we first created an instance of the EmployeeScheduler class. Then, we set the personal access token using set_token() method. After that, we built the DQM object using build_dqm() method. Then, we ran the DQM on the designated sampler using solve_problem() method. Finally, we printed the first solution to the employee scheduling problem using print_schedule() method.
Please note this Python program uses the D-Wave hybrid quantum/classical solver to solve a scheduling problem.