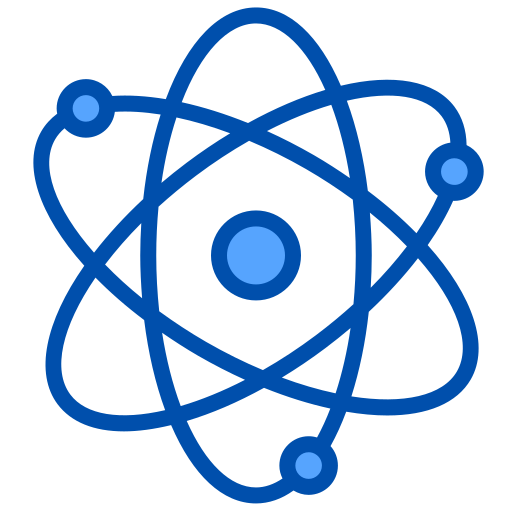
Maximum Heat by Quantum Annealing
Tatsuya Matsuda and Yoshiyuki Nakamura first proposed quantum annealing in 1998. They suggested that a quantum computer could solve optimization problems by exploiting the properties of quantum tunneling.
Quantum annealing is a quantum computing technique used to solve optimization problems. It is based on the physics of quantum tunneling, which allows particles to cross energy barriers and reach their lowest energy state. This is analogous to the process of annealing in classical materials science, where a material is heated and cooled at a controlled rate to reach its most stable state. Quantum annealing uses quantum tunneling to “hop” over local energy barriers and reach its global optimum solution faster than classical annealing. It is an iterative process that starts with a random solution and slowly refines it until the global optimum is reached.
QUBO stands for Quadratic Unconstrained Binary Optimization. An optimization problem involves minimizing a quadratic function of binary variables. QUBOs can be used to solve many optimization problems, such as those involving graph coloring, clique finding, and many others.
Building a QUBO for a problem is a multi-step process. First, you need to define the problem by writing down the objective function, constraints, and variables. The objective function should be a quadratic function of the binary variables. The constraints should be linear equations or inequalities involving the variables.
Next, you need to represent the problem in matrix form. To do this, you must create a matrix containing the coefficients of the objective function and the constraint equations. This matrix should have the same number of rows as the number of constraint equations and the same number of columns as the number of variables.
Once the problem is represented in QUBO matrix form, you can then map it to a quantum annealing machine to get the solution for your problem.
On the other hand, an Ising model is a powerful tool for tackling complex problems, as it is based upon the mathematical concept of Ising spins. These spins represent the states of a two-level quantum system and allow for encoding a problem space in terms of -1 and 1 variables. Furthermore, the Ising model is invaluable in optimizing the energy of a system, as well as finding the most advantageous configuration of a system. This makes it an ideal choice when attempting to maximize the performance of a quantum computer.
In the end, after the problem is solved, you can use the solution to find the optimal values for the variables. These optimal values can then be used to solve the original problem.
Problem:
Which switches do you need to flip to produce the maximum amount of heat?
You traced the wiring and found that one switch is wired to a heater producing 2W of heat, the other to a heater producing 3W, and both switches are wired to a cooler that drains 8W of heat when both switches are on.
To solve this problem using a QUBO, first, you set it up as a polynomial:
h = f(x_1,x_2) = 2x_1 + 3x_2 – 8x_1x_2Where h stands for the Hamiltonian of the system that we are trying to maximize here. As explained above, the next step is to map binary variables into Ising spin. To convert the binary available to Ising, we use below formula:
x_i = \frac{1-z_i}{2}After some algebraic simplifications, here is the Ising model that we want to pass to the quantum annealing machine to solve:
f(z_1,z_2) = \frac{1}{2} + z_1 + \frac{z_2}{2} – 2z_1z_2Caveat:
Please note that the annealing process always gives the ground state of the Hamiltonian of your system. To get the maximum Hamiltonian of the system, you should multiply the Ising formula by a -1, which yields to:
f(z_1,z_2) = \frac{1}{2} + z_1 + \frac{z_2}{2} – 2z_1z_2Alright! Now we should be good to go with our coding.
import pennylane as qml
from pennylane import numpy as np
H = - 1/2 * qml.Identity(1) - \
qml.PauliZ(1) - \
0.5 * qml.PauliZ(2) + \
2 * qml.PauliZ(1) @ qml.PauliZ(2)
print(H)
dev = qml.device("default.qubit", wires = H.wires)
@qml.qnode(dev)
def circuit(params):
for param, wire in zip(params, H.wires):
qml.RY(param, wires = wire)
return qml.expval(H)
circuit([1,1])
params = np.random.rand(len(H.wires))
opt = qml.AdagradOptimizer(stepsize = 0.5)
epochs = 100
for epoch in range(epochs):
params = opt.step(circuit, params)
print(f"hamiltonian of the system is {-circuit(params)} watt.")
dev = qml.device("default.qubit", wires = H.wires, shots = 1)
@qml.qnode(dev)
def circuit(params):
for param, wire in zip(params, H.wires):
qml.RY(param, wires = wire)
return qml.sample()
circuit(params)
Result:
By running the code, the possible answer to the solution is [0,1]. That means the circuit would generate 3 watts of heat if you set the X1 switch to OFF and the X2 to ON. The 3-watt is given by printing the circuits(params).